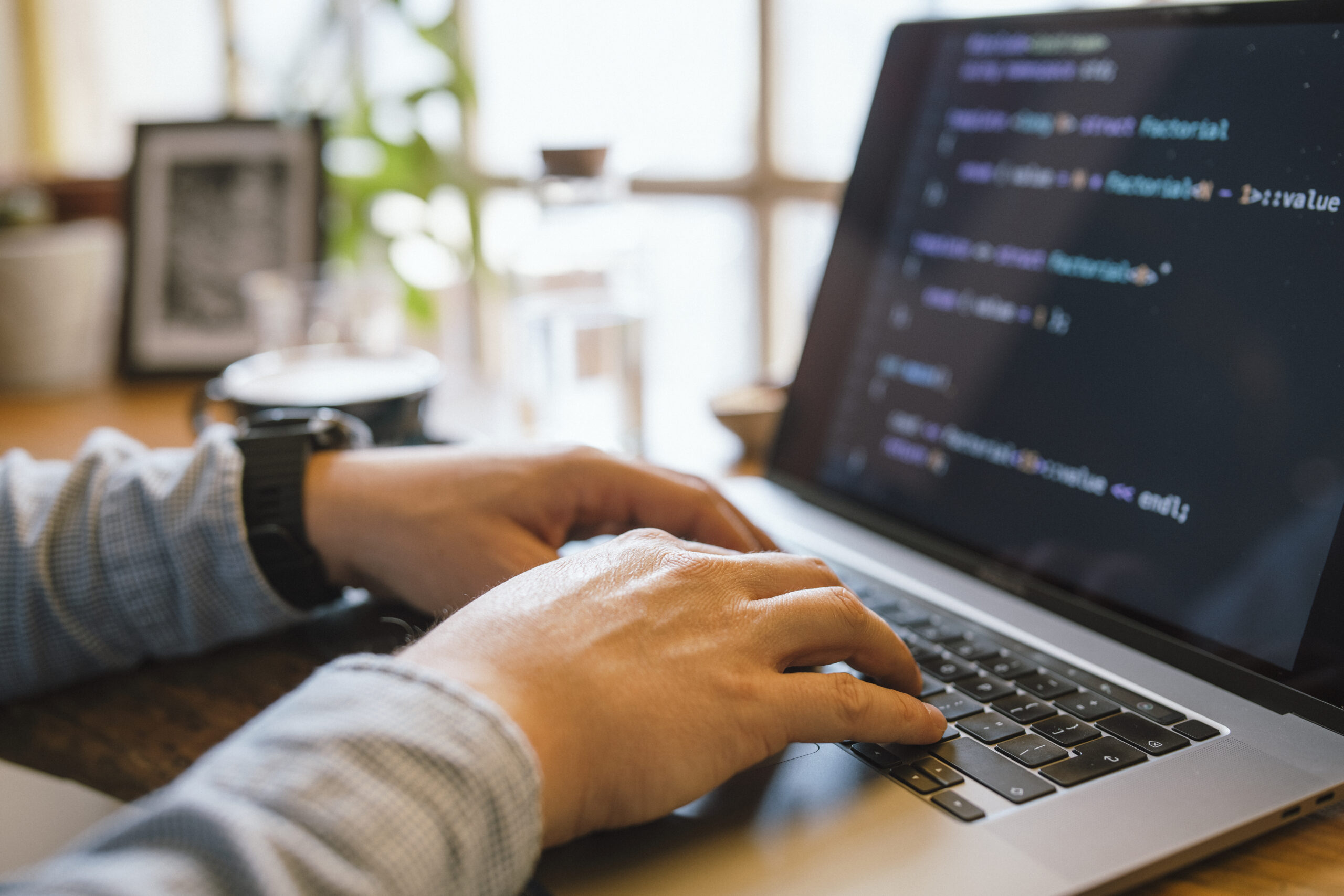
Debugging is Probably the most crucial — still normally ignored — capabilities in a very developer’s toolkit. It's not just about repairing damaged code; it’s about being familiar with how and why things go Incorrect, and Studying to Feel methodically to unravel complications competently. Whether or not you're a beginner or a seasoned developer, sharpening your debugging capabilities can preserve hrs of stress and considerably transform your productiveness. Here i will discuss quite a few procedures that will help builders degree up their debugging recreation by me, Gustavo Woltmann.
Grasp Your Equipment
One of many quickest ways builders can elevate their debugging techniques is by mastering the equipment they use on a daily basis. When composing code is a single A part of development, recognizing how to connect with it properly in the course of execution is equally significant. Present day progress environments arrive equipped with highly effective debugging capabilities — but many builders only scratch the surface area of what these tools can perform.
Get, for example, an Built-in Improvement Ecosystem (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These resources assist you to set breakpoints, inspect the worth of variables at runtime, phase through code line by line, and even modify code to the fly. When utilized the right way, they Allow you to notice specifically how your code behaves all through execution, which happens to be priceless for tracking down elusive bugs.
Browser developer resources, for instance Chrome DevTools, are indispensable for entrance-conclusion builders. They assist you to inspect the DOM, check network requests, see genuine-time general performance metrics, and debug JavaScript in the browser. Mastering the console, sources, and community tabs can transform annoying UI issues into manageable jobs.
For backend or procedure-degree builders, resources like GDB (GNU Debugger), Valgrind, or LLDB offer you deep Command more than managing procedures and memory management. Discovering these resources could possibly have a steeper Discovering curve but pays off when debugging overall performance troubles, memory leaks, or segmentation faults.
Further than your IDE or debugger, turn into at ease with Variation Manage techniques like Git to be aware of code record, discover the precise instant bugs were being introduced, and isolate problematic modifications.
In the end, mastering your equipment signifies going past default settings and shortcuts — it’s about building an intimate understanding of your growth natural environment to ensure that when problems come up, you’re not misplaced at midnight. The higher you understand your instruments, the greater time you may shell out fixing the particular difficulty as an alternative to fumbling as a result of the procedure.
Reproduce the situation
One of the more important — and sometimes neglected — measures in productive debugging is reproducing the situation. In advance of jumping in to the code or making guesses, builders will need to produce a reliable setting or situation exactly where the bug reliably seems. Devoid of reproducibility, repairing a bug gets a recreation of opportunity, normally resulting in wasted time and fragile code variations.
Step one in reproducing a dilemma is collecting as much context as is possible. Request questions like: What steps resulted in the issue? Which environment was it in — progress, staging, or manufacturing? Are there any logs, screenshots, or mistake messages? The more element you might have, the less complicated it gets to be to isolate the precise situations less than which the bug happens.
Once you’ve gathered enough information, endeavor to recreate the issue in your neighborhood atmosphere. This may imply inputting the exact same facts, simulating comparable consumer interactions, or mimicking system states. If The problem seems intermittently, think about producing automated checks that replicate the edge situations or point out transitions involved. These assessments don't just assist expose the problem but in addition reduce regressions Later on.
From time to time, The difficulty might be atmosphere-particular — it would materialize only on particular working devices, browsers, or under specific configurations. Using resources like virtual devices, containerization (e.g., Docker), or cross-browser tests platforms is usually instrumental in replicating such bugs.
Reproducing the trouble isn’t merely a step — it’s a attitude. It calls for endurance, observation, in addition to a methodical approach. But when you can constantly recreate the bug, you might be already halfway to fixing it. With a reproducible scenario, You can utilize your debugging equipment a lot more successfully, check possible fixes securely, and talk much more clearly together with your group or people. It turns an summary grievance into a concrete challenge — and that’s where builders prosper.
Browse and Understand the Mistake Messages
Mistake messages are sometimes the most useful clues a developer has when anything goes Mistaken. In lieu of observing them as annoying interruptions, developers ought to learn to take care of mistake messages as direct communications through the technique. They usually tell you what precisely took place, in which it occurred, and sometimes even why it happened — if you know the way to interpret them.
Start out by reading through the message diligently As well as in complete. Lots of builders, particularly when below time pressure, look at the very first line and straight away start out producing assumptions. But deeper during the error stack or logs may lie the genuine root result in. Don’t just duplicate and paste error messages into search engines like google — study and have an understanding of them very first.
Crack the error down into pieces. Could it be a syntax mistake, a runtime exception, or even a logic mistake? Does it point to a certain file and line number? What module or operate brought on it? These queries can guideline your investigation and level you toward the dependable code.
It’s also helpful to grasp the terminology of the programming language or framework you’re employing. Mistake messages in languages like Python, JavaScript, or Java frequently observe predictable designs, and Discovering to recognize these can substantially increase your debugging method.
Some glitches are imprecise or generic, and in those circumstances, it’s important to look at the context by which the error happened. Check connected log entries, enter values, and recent adjustments from the codebase.
Don’t ignore compiler or linter warnings either. These usually precede much larger challenges and provide hints about likely bugs.
Finally, mistake messages aren't your enemies—they’re your guides. Understanding to interpret them effectively turns chaos into clarity, encouraging you pinpoint issues quicker, minimize debugging time, and turn into a extra efficient and confident developer.
Use Logging Wisely
Logging is Just about the most strong equipment in a very developer’s debugging toolkit. When applied successfully, it provides genuine-time insights into how an application behaves, assisting you comprehend what’s happening under the hood without needing to pause execution or step throughout the code line by line.
An excellent logging method begins with realizing what to log and at what degree. Typical logging ranges include DEBUG, Data, WARN, ERROR, and Lethal. Use DEBUG for specific diagnostic facts for the duration of growth, Data for common functions (like productive begin-ups), Alert for probable difficulties that don’t split the application, Mistake for genuine complications, and Deadly once the system can’t go on.
Prevent flooding your logs with extreme or irrelevant information. Too much logging can obscure significant messages and decelerate your program. Concentrate on vital gatherings, state variations, enter/output values, and critical final decision factors within your code.
Structure your log messages Plainly and regularly. Involve context, for example timestamps, request IDs, and function names, so it’s much easier to trace troubles in distributed systems or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
Through debugging, logs let you observe how variables evolve, what circumstances are fulfilled, and what branches of logic are executed—all with out halting This system. They’re Specially valuable in creation environments where stepping by way of code isn’t possible.
Furthermore, use logging frameworks and applications (like Log4j, Winston, or Python’s logging module) that help log rotation, filtering, and integration with checking dashboards.
Eventually, clever logging is about balance and clarity. By using a well-believed-out logging tactic, you are able to decrease the time it's going to take to spot difficulties, gain deeper visibility into your apps, and Increase the overall maintainability and dependability of your respective code.
Think Like a Detective
Debugging is not merely a technological job—it's a sort of investigation. To correctly determine and resolve bugs, builders ought to approach the method similar to a detective resolving a secret. This mindset assists break down intricate difficulties into workable pieces and stick to clues logically to uncover the basis bring about.
Start out by accumulating proof. Think about the indications of the problem: error messages, incorrect output, or overall performance troubles. The same as a detective surveys against the law scene, accumulate just as much suitable facts as you could without the need of leaping to conclusions. Use logs, take a look at scenarios, and person experiences to piece alongside one another a transparent photo of what’s occurring.
Following, kind hypotheses. Request oneself: What could possibly be triggering this conduct? Have any adjustments not too long ago been produced to the codebase? Has this issue happened in advance of beneath equivalent conditions? The aim would be to slender down options and discover prospective culprits.
Then, test your theories systematically. Seek to recreate the situation in the controlled atmosphere. If you suspect a certain operate or component, isolate it and validate if the issue persists. Similar to a detective conducting interviews, question your code concerns and Enable the outcome direct you nearer to the truth.
Pay shut consideration to tiny details. Bugs generally conceal in the least predicted locations—similar to a missing semicolon, an off-by-just one error, or maybe a race problem. Be complete and individual, resisting the urge to patch The difficulty without the need of completely understanding it. Short term fixes may conceal the actual issue, just for it to resurface later.
And finally, continue to keep notes on That which you tried and uncovered. Equally as detectives log their investigations, documenting your debugging method can help save time for long term troubles and assistance Other individuals have an understanding of your reasoning.
By considering just like a detective, builders can sharpen their analytical skills, strategy challenges methodically, and turn out to be simpler at uncovering concealed challenges in complicated techniques.
Produce Checks
Composing assessments is among the simplest methods to increase your debugging techniques and overall improvement effectiveness. Exams not simply enable catch bugs early but additionally serve as a safety Internet that provides you self esteem when building variations to your codebase. A nicely-tested application is simpler to debug as it means that you can pinpoint accurately where by and when a dilemma takes place.
Get started with device checks, which center on unique capabilities here or modules. These small, isolated tests can quickly expose whether a selected bit of logic is Performing as predicted. Each time a examination fails, you right away know in which to seem, drastically lowering time spent debugging. Device checks are In particular valuable for catching regression bugs—concerns that reappear following previously remaining fixed.
Future, combine integration exams and end-to-close assessments into your workflow. These aid make sure that various portions of your application function alongside one another effortlessly. They’re specially beneficial for catching bugs that occur in elaborate programs with numerous factors or companies interacting. If some thing breaks, your checks can let you know which part of the pipeline unsuccessful and beneath what conditions.
Producing tests also forces you to definitely Believe critically regarding your code. To test a element effectively, you need to be aware of its inputs, expected outputs, and edge scenarios. This amount of understanding Obviously prospects to higher code structure and less bugs.
When debugging a difficulty, creating a failing take a look at that reproduces the bug can be a strong starting point. Once the exam fails constantly, you could concentrate on repairing the bug and check out your check move when The difficulty is resolved. This strategy makes certain that the same bug doesn’t return Later on.
Briefly, composing assessments turns debugging from the irritating guessing recreation right into a structured and predictable system—assisting you catch far more bugs, a lot quicker and much more reliably.
Get Breaks
When debugging a difficult challenge, it’s straightforward to become immersed in the situation—gazing your screen for hours, attempting Remedy soon after Option. But one of the most underrated debugging tools is simply stepping away. Taking breaks will help you reset your head, lower irritation, and infrequently see The difficulty from the new standpoint.
If you're much too near the code for far too extensive, cognitive exhaustion sets in. You may perhaps get started overlooking apparent mistakes or misreading code that you simply wrote just hours earlier. In this point out, your Mind gets considerably less productive at difficulty-solving. A short wander, a espresso crack, or maybe switching to a unique process for 10–15 minutes can refresh your aim. Quite a few builders report locating the root of a problem when they've taken time and energy to disconnect, letting their subconscious work within the background.
Breaks also assistance protect against burnout, Specially in the course of longer debugging classes. Sitting in front of a display screen, mentally caught, is not only unproductive but will also draining. Stepping away allows you to return with renewed Electrical power in addition to a clearer way of thinking. You could suddenly detect a missing semicolon, a logic flaw, or simply a misplaced variable that eluded you ahead of.
In the event you’re trapped, a great general guideline is always to established a timer—debug actively for 45–sixty minutes, then take a five–10 moment break. Use that point to move all over, stretch, or do a thing unrelated to code. It may sense counterintuitive, Particularly less than tight deadlines, but it surely really brings about speedier and more effective debugging Eventually.
In short, using breaks is not really a sign of weak point—it’s a sensible technique. It offers your Mind Area to breathe, enhances your standpoint, and assists you stay away from the tunnel eyesight That usually blocks your development. Debugging is a mental puzzle, and relaxation is an element of solving it.
Find out From Just about every Bug
Every bug you come across is a lot more than simply a temporary setback—It really is a chance to mature as being a developer. Irrespective of whether it’s a syntax error, a logic flaw, or perhaps a deep architectural situation, every one can instruct you something useful in case you go to the trouble to replicate and analyze what went Incorrect.
Commence by inquiring on your own a handful of key concerns when the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught before with better practices like unit testing, code reviews, or logging? The answers often reveal blind places in the workflow or understanding and help you build stronger coding habits shifting forward.
Documenting bugs may also be a great behavior. Maintain a developer journal or maintain a log in which you Take note down bugs you’ve encountered, the way you solved them, and That which you uncovered. After a while, you’ll start to see patterns—recurring issues or popular faults—you can proactively keep away from.
In staff environments, sharing That which you've realized from a bug with your friends might be Particularly powerful. Whether it’s via a Slack concept, a brief produce-up, or a quick knowledge-sharing session, encouraging Other folks avoid the exact challenge boosts staff effectiveness and cultivates a much better Finding out tradition.
Extra importantly, viewing bugs as lessons shifts your mindset from annoyance to curiosity. As opposed to dreading bugs, you’ll start appreciating them as necessary elements of your enhancement journey. All things considered, a few of the finest developers are certainly not the ones who produce best code, but those that repeatedly discover from their faults.
In the end, Every single bug you fix provides a completely new layer in your talent set. So following time you squash a bug, have a moment to mirror—you’ll occur away a smarter, far more able developer due to it.
Summary
Enhancing your debugging techniques takes time, follow, and tolerance — but the payoff is big. It would make you a far more effective, assured, and able developer. Another time you're knee-deep within a mysterious bug, recall: debugging isn’t a chore — it’s a possibility to become far better at Whatever you do.